JSP: Select and Process Table Row Data
May 14, 2014
1. Introduction
This is a hotel room reservation web application example. This shows the selection and processing of table row data. The application uses Java Servlet and JavaServer Pages (JSP) technology.
The hotel clerk takes a call from a guest and enters initial room selection criteria and submits a search. The resulting selection form displays 5 to 10 available hotel rooms as table rows. Based on the guest's need the clerk selects a room (or rooms) and submits it for reservation process and a confirmation.
A JSP page displays a table with rows of available rooms in the room selection form. In the web application, a row can be selected using a radio button (for single selection), or check boxes (for multiple selections). Then submit the data by clicking the submit process button.
In addition to the above two selection cases, there is a 3rd case to use a submit button (or a link) on each row.
1.1. About
This post is an example with code snippets as used in JSP for the above mentioned three cases for selecting and processing table row(s) data:
- Case 1: Using Radio Button to Select Single Row Data
- Case 2: Using Checkboxes to Select Multiple Row Data
- Case 3: Using Submit Button to Select & Submit Single Row Data
NOTE: It is possible to use an input textbox to enter the selected row number of the table row in the room selection form and submit for reservation. The table rows have a row number column. Multiple rows can be selected by entering comma separated row number values in the input textbox.
2. Room Search form:
This is the application's initial form (this form's screenshot is not shown in this post). The user enters room selection criteria like, reservation from date, room type, is smoking and submits the search. The search logic gets the available rooms and displays them in the following Room Selection form.
A List
collection (of type HotelRoom
with attributes no, type, smoking, rate
and availableDate
) has the available rooms from the search. This is stored as a session attribute (or session scoped variable) named availableRooms
.
3. Case 1: Using Radio Button to Select the Row Data
3.1. Room Selection form:
In the page the search result data is displayed in rows using JSTL tags with data from the session attribute availableRooms
. Each row is displayed with a radio button as a column value.
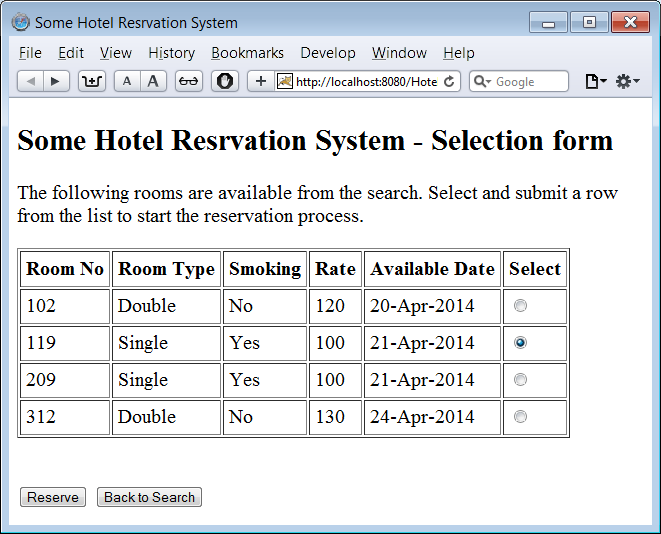
The following is the code snippet to display the available rooms:
<c:forEach var="room" items="${sessionScope.availableRooms}"
varStatus="status">
${room.no}
...
<input type="radio" name="radioButton" value="${status.count}">
</c:forEach>
The value
attribute of radio button has the ${status.count}
value. This is the current round of iteration (and this starts from 1, not zero).
The user selects a table row by clicking the radio button. Then submits the form by clicking the Reserve button; and this action takes to the Reservation form.
Note that only one row can be processed on submitting the form, i.e., only one room can be reserved by the clerk at a time.
3.2. Reservation form:
The selected row index in the Room Selection form is available in this form as a request parameter (name radioButton
) value. Get the parameter value and reduce its value by 1 (The availableRooms
is a List
collection and its index starts from zero. The parameter value has the table iteration count starting from 1. To get the correct List
index from displayed row, reduce the parameter value by 1):
<c:set var="rowno" value="${param.radioButton - 1}"/>
Use the rowno
page variable to get the row data values from the session scoped variable availableRooms
.
The following is the code snippet to show the selected row data:
${sessionScope.availableRooms[rowno].no}
${sessionScope.availableRooms[rowno].type}
${sessionScope.availableRooms[rowno].smoking}
${sessionScope.availableRooms[rowno].rate}
${sessionScope.availableRooms[rowno].availableDate}
NOTE: The param
, paramValues
and sessionScope
are the JSP EL implicit objects for request parameter, request parameters and session scope respectively.
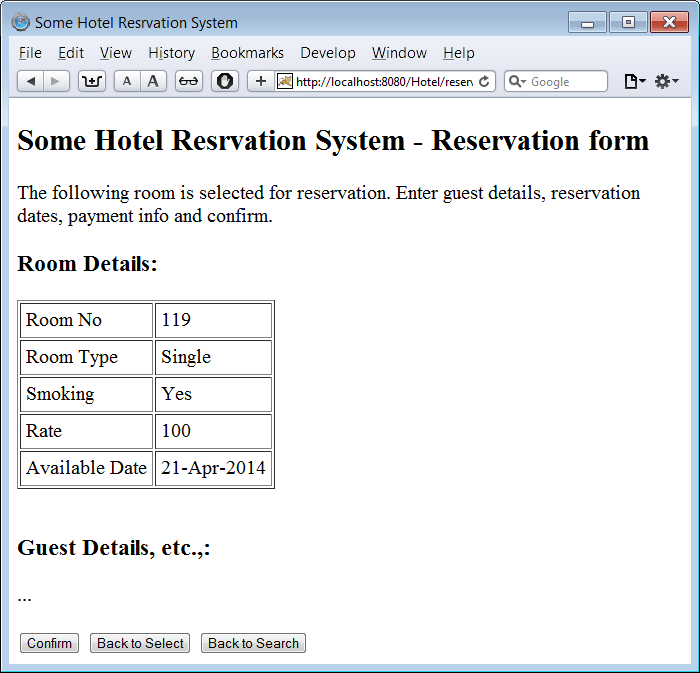
Details to complete the reservation process are entered (guest and payment info, reservation dates etc.,) and the form is submitted for the reservation confirmation.
The Confirmation form shows the hotel room reservation confirmation details (this form's screenshot is not shown in this post).
4. JSP Coding:
HTML, JSP EL (Expression Language) and JSTL (JSP Tag Library) core tags are used in coding the JSP pages.
4.1. Source Code
Click this link to view the JSP code for the Room Selection and the Reservation forms for the Case 1. (Note that the code for the following is not included in this post, but can be downloaded: (1) Case 2 and Case 3, and (2) the servlet code to start the application and load the hotel room data from a database, the initial Room Search (search criteria entry and submit) form and the Confirmation form.)
4.2. Download
Download source code here (for all the three cases): JSPSelectTableRowsExample.zip
Continue to Case 2 and Case 3 >>
Return to top